CSC120 Week 10 Lab.: Write-in Election
cs编程代写 The goal of this lab is to write a program, Election, that receives an indefifinite number of votes in a write-in election, tallies the votes…
Instructor: Mitsu Ogihara
The goal of this lab is to write a program, Election, that receives an indefifinite number of votes in a write-in election, tallies the votes received, and fifinds the winner.
The Main Method cs编程代写
The main method consists of three method calls.
initialize(); receive(); findWinner();
The three methods respectively (a) initialize the common data and describe the action of the program, (b) receive input, and (c) compute and report the winner.
Input Format
We assume that the votes arrive as pairs of lines. In each pair, the fifirst line is a name and the second line is a count for the name. Any name may appear multiple times. The end of the votes will be indicated by a single minus sign appearing as the name. Once the end marker appears, the program will ignore the rest of the input. For example, the input can be: cs编程代写
Anne 20 Barbara 30 Carol 20 Anne 25 Barbara 10 Dave 4 Dave 5 -
The total votes are 45 for Anne, 40 for Barbara, 20 for Carol, and 9 for Dave. Thus, the winner is Anne and the runner-up is Barbara. When more than one name has the largest votes, the program may claim any one among those as the winner. The winner selection process, which appears later in the document, automatically breaks a tie in this manner.
Static Variables
The program must use three static variables, String[] names, int[] counts, and Scanner keyboard. The fifirst two are for recording the names and the total votes, respectively, and the last is for receiving input from the keyboard. The two arrays will have the same lengths. For each position of the two arrays, the element of names at the position and the element of counts at the position record in a pair the name and its total votes. Initially, both arrays have length 0. The initialization of the two arrays occurs at the start of the main method.
The Input Format cs编程代写
The votes come in pairs of lines. The fifirst line of each pair is the name of a person, which may contain the white space in it The second line is the votes for the person. Let us say n is the String variable that receives the fifirst line. If the value of n happens to be equal to “-“, the while loop should terminate immediately. Otherwise, it receives the next line, stores in a variable, say w, and then using the method Integer.parseInt with w as its actual parameter to convert it to an int c. If this conversion is successful, we add the pair of information n and c to the record.
Otherwise, we ignore the pair. To accomplish this, we use the fact that the method Integer.parseInt produces a run-time error NumberFormatException when its parameter does not represent an integer. We put the actions of reading w, obtaining c, and adding the pair in a single try and attach a catch-block after it that produces an error message “Not an integer. Skip this pair.”. cs编程代写
Adding One Pair of Information
We use a method add( String n , int c ) for adding a pair of information. For adding the pair to the record, we fifirst check if the name n already appears in names. The method find receives a String parameter n and fifinds the position at which n appears in names. Following the idea in indexOf for String, the method returns -1 if n does not appear in names. The action of find is simple. It uses a for-loop over the valid indices on names to scan the array. As soon as it encounters an element equal to n (in terms of the String equality), the method returns that index. When the loop completes, we have learned that the name does not presently appear on the array. The method thus returns -1.
Using the method find, we can add n and c as follows:
- Call find( n ) to obtain its return value.
- If the returned value is >= 0, we update the element of counts at the position given by the returned value by adding c.
- Otherwise, we extend the two arrays by one element each and store the new values n and c, respectively to the array.
Finding the Winner cs编程代写
After receiving all the input, we compute the winner. The method findWinner, while reporting how many votes each person received, in the order of appearance in the array names, computes the winner. Specififically, the method uses two int variables, maxCount and theWinner. The initial value is 0 for both variables. The method scans the contents of the array using a for-loop with an iteration variable, say j. During the scan, if counts[ j ] is greater than maxCount, names[ j ] is the present winner, so the program updates maxCount with counts[ j ] and theWinner with j.
At the conclusion of the loop, the winner’s name is names[theWinner] with maxCount The program should report the winner-fifinding process on the screen and in a fifile named “results.txt”. The method findWinner instantiates a File object named f with “results.txt” as the actual parameter and then instantiates a PrintStream object named stream with f as the actual parameter. The latter instantiation may produce FileNotFoundException and so attach throws FileNotFoundException to both findWinner and main.
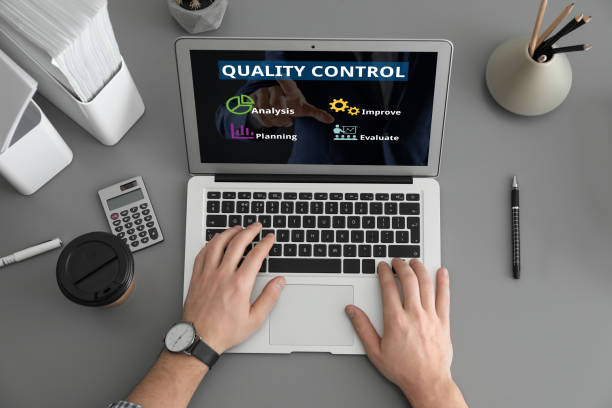
In the execution example below, the line starting with Results is the reporting. Duplicate each output action on stream. At the end of the method findWinner, execute stream.flush() and then stream.close() to ensure that the reporting into the fifile is complete.
########################################### # Enter data in pairs of lines with # # the name in first line and # # the count in the second line. # # End with -. # ########################################### Julia 10 Jackie 20 Johnny 30 Julia 23 Jackie 20 Joyce 25 Jeremy 35 Jackie 1 Jeremy 09g Not an integer. Skip this pair. - Results Julia 33 Jackie 41 Johnny 30 Joyce 25 Jeremy 35 - Jackie won.
The fifirst fifive lines are the description of the program. The line before the dashes is an error message triggered by a non-integer line 09g that follows the last appearance of Jeremy.