BME 1108A Project 4: COVID-19 Vaccine Distribution
Computer Science代写 Engineering intersections: Industrial & Operations Engineering / Computer Science; Healthcare Access Logistics…
Engineering intersections: Industrial & Operations Engineering / Computer Science; Healthcare Access Logistics
Implementing this project provides an opportunity to practice file I/O, functions, loops, and data structures (arrays and structs).
The program design is worth 10 points, the functionality portion of the final submission is worth 100 points, and the style and comments grade is worth 10 points.
You may work alone or with a partner. Please see the syllabus for partnership rules.
Project Roadmap Computer Science代写
This is a big picture view of how to complete this project. Most of the pieces listed here also have a corresponding section later on in this document that goes into more detail.
- Read through the project specification (DO THIS FIRST)
- Complete the Program Designand submit it to Moodle
Please do this first! I’ll ask to see it before I help you with any coding or debugging questions. 完成程序设计并提交给Moodle
请先这样做!在我帮助您解决任何编码或调试问题之前,我将要求您去看看它。
- Write helper functions to handle the data input part of the program
Get these parts working first; verify you are reading in the data correctly! 编写辅助程序函数来处理程序的数据输入部分
首先让这些部件工作,验证您读取的数据是否正确!
- Create the location gridand determine the route
To debug your loops, use printf statements to print some intermediate variable values to the command prompt; you can use these values to check your calculations. 创建位置网格并确定路由要调试循环,请使用printf语句将一些中间变量值打印到命令提示符;可以使用这些值来检查计算。
Once it’s working, delete the printf statements and put in the code to print to the output file. 工作后,删除printf语句并放入代码以打印到输出文件。
- Check that your program is sufficiently and correctly commented
- Verify that you can reproduce the Test Cases in the Appendix
- Submit cto Dr. E for testing
Background Computer Science代写
To put an end to the COVID-19 pandemic, it is very critical to vaccinate as much of the global population as possible with vaccines that have high efficacy and provide protection against several of the emerging variants of the SARS-CoV-2 virus. To complete our work this semester, you now have the task of proposing a vaccine delivery system in order to efficiently and cost-effectively supply the world’s leading COVID-19 vaccines to as many people as possible. While logistics generally fall under the purview of industrial engineers, Dr. E has a wild idea. DiDi is an already well-established and efficient transportation network. What if we align our network of vaccine delivery systems with those of existing ride-share companies, such as DiDi?! Thus, we will partner with DiDi to form DiDiVaccine™!
The DiDi database has a list of coordinates corresponding to common rideshare locations in a few countries, but we will expand this system to the rest of the world for the delivery of our product. Note: other rideshare companies, such as Lyft and Uber, are already providing free rides for people to vaccination sites and may provide additional information to help us in our goal, as aiding the vaccination effort is good for company morale/public relations and the greater good of the human race (and also the long-term bottom line profits of the company).
Expanding DiDi’s service requires a computer program that will automatically plan routes based on the shortest path through the customers’ destinations. Each driver is restricted to their own range within a distribution center built by the vaccine companies (more on this in Part II), so the program must limit the destinations to only those locations within a maximum area defined by a grid. Several grids will be placed around the world.
Your Job
In this project, your job is to find the shortest path from each vaccination site to the next within your DiDi driver’s range. 在这个项目中,您的任务是在您的滴滴司机的范围内找到从每个接种点到下一个接种点的最短路径。
Engineering Concepts
There are two high-level engineering concepts that will be used in this project: the traveling
salesperson problem and data corruption. These two terms are briefly explained in the next sections.
Traveling Salesperson Problem (TSP)
The traveling salesperson problem is a commonly-used optimization problem that asks,
Given a list of cities and the distances between each pair of cities, what is the shortest possible route that visits each city exactly once and returns to the origin city? [Wikipedia] 给出城市列表和每一对城市之间的距离,恰好访问每个城市一次并返回原始城市的最短的路线是什么?[维基百科]
The “cities” and “distances” can have different definitions according to the application of the TSP algorithm. The problem has analogous questions in circuit design (shortest distance the laser needs to travel to create a circuit board – this also applies to efficient use of a laser cutter, like in the STU-BME makerspace!), delivery logistics (shortest time to complete package delivery to homes and/or businesses), school bus routes, and similar engineering challenges.
There are many different algorithms that have been developed to solve this problem. In this project, we will use the nearest neighbor algorithm to determine which vaccination site to go to next. 根据TSP算法的应用,“城市”和“距离”可以有不同的定义。这个问题在电路设计中也有类似的问题(激光创建电路板需要走的最短距离来创建一个电路板——这也适用于激光切割器的有效使用,比如在STU-BME制造空间中!)、送货物流(完成到家庭和/或企业的包裹送货的最短时间)、校车路线以及类似的工程挑战。
有许多不同的算法已经被开发来解决这个问题。在这个项目中,我们将使用最近邻算法来确定下一个要去哪个疫苗接种地点。
The nearest neighbor algorithm we will use is defined here as:
- Start at a given location (distribution center A)
- Find the closest vaccination site that has not already been visited
- Go to that location
- Track that this location has been “visited”
- Repeat steps 2-4 until all vaccination sites have been visited
- Go to the specified end location (distribution center B)
从给定位置开始(配送中心A)
找到最近的尚未访问的疫苗接种站点
前往那个位置
跟踪该位置已被“访问”
重复步骤2至4,直到访问了所有疫苗接种地点
进入指定的结束位置(配送中心B)
A sample path found using this algorithm is shown in Fig. 2 (click the link to see the path animated). Note that this is a “greedy” algorithm — it picks the single closest location that hasn’t already been visited, regardless of where the other yet-to-be-visited locations are, traffic in and out of those locations, or total distance traveled. Therefore, this greedy algorithm does not generally find the best path through all the required locations, but it often finds a path that is close to optimal. Step 6 above is required for our particular DiDiVaccine™ application — it is not a necessary part of the nearest neighbor algorithm.
使用此算法找到的样本路径如图所示。2(单击链接以查看动画路径)。请注意,这是一种“贪婪”算法——它选择尚未访问的最近位置,无论其他待访问的位置在哪里、进出这些位置的交通或旅行的总距离。因此,这种贪婪算法通常不会找到通过所有所需位置的最佳路径,但它通常会找到一条接近最优的路径。上面的步骤6是我们的特定的直接接种疫苗™应用程序所必需的——它不是最近邻算法的必要部分。
Figure 1. Example of how the nearest neighbor algorithm works. Note how the path changes based on the starting location!
(This is a .gif so please do click the source (also available in PPT slide posted to Moodle: Photo Source)
To determine which new location is “closest” to the current location, we will assume that the vaccination sites all lie in roughly the same plane, despite being on the curved surface of the Earth. Think of this as a 2-D map. Thus, we can use Cartesian coordinates and the following formula for distance: 为了确定哪个新位置“最接近”当前位置,我们将假设疫苗接种地点都位于大致相同的平面上,尽管它们位于地球的曲面上。你可以把它看作是一张二维地图吧。因此,我们可以使用笛卡尔坐标和以下距离公式:
_______________________________________
distance = √(xcurrent − xpotential)2 + (ycurrent − ypotential)2
where xcurrent and ycurrent are the coordinates of the current location and xpotential and ypotential are the coordinates of the next location to possibly travel to.其中xcurent和ycurert是当前位置的坐标,xbecent和y电势是可能到达的下一个位置的坐标。
Data Corruption 数据腐烂
Transmission of data is subject to potential corruption. Data corruption occurs when data from one computer is sent to another computer and the data received by the second computer does not match what was sent by the first computer. Data are stored in computers in binary form: a bunch of 0s and 1s. When this data gets sent from one computer to another, it is possible for a 0 to get flipped to a 1, and vice-versa, due to noise in the system. This means the data are corrupted and need to be fixed. There are many ways of checking for data corruption; error detection and correction is a critical component of modern computing. In this project, we will implement a simple error detection and correction based on known error effects. See below for further information. 数据的传输可能存在潜在的损坏。当来自一台计算机的数据被发送到另一台计算机,且第二台计算机接收到的数据与第一台计算机发送的数据不匹配时,就会发生数据损坏。数据以二进制形式存储在计算机中:一组0s和1s。当这些数据从一台计算机发送到另一台计算机时,由于系统中的噪声,0有可能被翻转到1,反之亦然。这意味着数据已损坏,需要进行修复。检查数据腐败的方法有很多;错误检测和校正是现代计算的一个关键组成部分。在本项目中,我们将实现一个基于已知误差效应的简单错误检测和修正。有关更多信息,请见下文。
Program Tasks
For this project, you need to determine the shortest route for your DiDiVaccine™ driver. The route starts from a given starting point (distribution center), proceeds to the vaccination sites, and ends at the given endpoint (another distribution center, perhaps the same one, perhaps not). You will need to read in data from input files, determine the route, and create an output file with a “map” of the vaccination sites and the route the DiDiVaccine™ driver needs to take to get from the starting point to the end point. These tasks are detailed in the next sections. 对于此项目,您需要确定您的直接接种疫苗™驱动程序的最短路线。路线从一个给定的起点(分发中心)开始,继续到疫苗接种地点,最后到达给定的终点(另一个分发中心,可能是同一中心,可能不是)。您需要从输入文件中读取数据,确定路线,并创建疫苗™驱动程序从起点到终点的输出文件。下几节将详细介绍这些任务。
Input Data
There are two input files that are required by your program: 1) a locations file, and 2) a vaccination site names file. The files themselves are defined in the Input Files Section. The sections below describe the procedures needed to process the data within the input files. 您的程序需要两个输入文件:1)一个位置文件和2)一个疫苗接种站点名称文件。这些文件本身将在“输入文件”部分中进行定义。以下部分介绍了处理输入文件中的数据所需的过程。
Get Filenames
Your program must prompt the user for the names of the two input files (this makes the program flexible — the person using it can call the files whatever they want). Prompt the user for the locations file first using “Enter Locations Filename: ”; the user then enters the <location_file>.txt name. Then, prompt the user for the vaccination names filename, <name_file>.txt with “Enter Names Filename: ”. 程序必须提示用户输入两个输入文件的名称(这使得程序更加灵活——使用它的人可以调用他们想要的文件)。首先使用“输入位置文件名:”提示用户输入位置文件,然后用户输入<location_file>。txt名称。然后,用“输入名称文件名:”提示用户输入疫苗接种名称文件名<name_file>。txt。
Open Files
Once both input filenames have been entered by the user, the program must then open the files for reading. If either of the input files is not found or cannot be opened for reading, the program must output “Input file could not be opened” to the command prompt, followed immediately by (on a separate line) program exit with code return 1;. Note that you do not need to determine which file caused the failure; simply print the message and exit the program. Refer to the lecture notes for a refresher on how to check for inability to read files. 一旦用户输入了两个输入文件名,程序就必须打开这些文件进行读取。如果找不到输入文件或无法打开读取,程序必须将“输入文件无法打开”输出到命令提示符,然后立即(在另一行)程序退出,代码返回1;。请注意,您不需要确定是哪个文件导致了故障;只需打印消息并退出程序。有关如何检查是否无法读取文件,请参阅课堂讲稿。
Note
The provided test cases do not test the error handling of input files. You must generate your own test case to be sure you meet this requirement. Do not submit your test case, just ensure your code satisfies this important project specification. 所提供的测试用例不测试输入文件的错误处理。您必须生成您自己的测试用例,以确保您满足此要求。不要提交测试用例,只需确保您的代码满足这个重要的项目规范。
Read Data
The locations file and the vaccination site names file are detailed in the Input Files section. Note that the locations file contains the range for that particular DiDiVaccine™ driver (i.e., number of rows and columns in their “map”), the starting and ending points for the journey, and the data for the vaccination sites. The names of the vaccination sites are stored separately in the names file. Input all the location and names data, and appropriately store the information for further use (you choose the storage method). 位置文件和疫苗接种地点名称文件的详细信息在输入文件部分。请注意,位置文件包含特定DiDi疫苗™驱动程序的范围(即其“地图”中的行数和列数)、旅程的起点和终点以及疫苗接种站点的数据。疫苗接种位点的名称分别存储在名称文件中。输入所有位置和名称数据,并适当地存储信息以供进一步使用(请选择存储方法)。
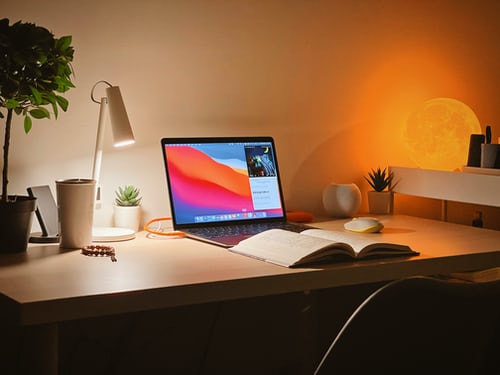
Recommendation: use structs!
We highly recommend that you use an array of structs to store the vaccination site data! See the Week 16 lecture notes on structs for inspiration. 建议:使用结构体!
我们强烈建议您使用一组结构来存储疫苗接种站点数据!灵感请参见第16周的结构课堂讲稿。
As you check each vaccination site in the file, you must ensure that its coordinates lie within the grid – if they do not, your code must output “<Vac Site ID> out of range – ignoring” to the terminal, where <Vac Site ID> is the ID of the vaccination site whose coordinates are not within the grid. 检查文件中的每个疫苗接种站点时,必须确保其坐标位于网格内—如果不存在,代码必须输出“<Vac站点ID>超出范围-忽略”到终端,其中<Vac站点ID>是坐标不在网格内的疫苗接种站点的ID。
Correct Errors
Please look at the names files for the test cases. You will notice that some of the vaccination site names have been corrupted during file transmission: there are “YY”s that have been erroneously added into the vaccination site names. You need to: 请查看测试用例的名称文件。您会注意到一些疫苗接种站点名称在文件传输过程中已损坏:有些“YY”是被错误地添加到疫苗接种站点名称中的“YY”。您需要:
- Find and erase occurrences of “YY” from each name
- Find and replace all of the underscores with spaces.
- 查找并从每个名称中删除“YY”的事件
- 查找并用空格替换所有的下划线。
Create Location Grid
As part of the output file, route.txt, you must provide a grid of characters representing the “map” of locations within your DiDiVaccine™ driver’s range. The locations file indicates the number of rows and columns that must appear in the grid. The locations file also contains a 1-character symbol representing that vaccination site. 作为输出文件route.txt的一部分,必须提供表示DiDiViccine™驱动程序范围内位置“映射”的字符网格。位置文件指明必须显示在网格中的行数和列数。位置文件还包含一个表示该疫苗接种站点的1个字符的符号。
Place each vaccination site’s symbol at its corresponding grid location (row, column). If a map location is not represented by a vaccination site symbol, then place a period (“.”) at that grid point. Also, place an “S” at the starting point and an “E” at the ending point. 将每个疫苗接种站点的符号放在其相应的网格位置(行、列)。如果地图位置未用疫苗接种站点符号表示,则在该网格点处放置一个周期(“。”)。同时,在起点放置“S”,在终点放置“E”。
Once you have created your grid point map, output the map to file route.txt. 创建网格点图后,将映射输出到文件route.txt。
Watch your indexing!
On your grip point map, input row and column values start from 1, not 0. Row numbers increase from the top row to the bottom row and column numbers increase from the left column to the right column. In other words, the upper left corner of the grid map corresponds to (row 1, column 1). Note that if you store your map in a 2-D array, C array indices begin with index 0. Check, then double check your indexing!在抓地点贴图上,输入行和列值从1开始,而不是从0开始。行数从上行增加到下行,列号从左列到右列增加。换句话说,网格图的左上角对应于(第1行,第1列)。请注意,如果将映射存储在二维数组中,C数组索引将以索引0开头。检查一下,然后再次检查一下你的索引!
Determine the Route
Acting as dispatcher for DiDiVaccine™ drivers, implement the nearest neighbor algorithm. To determine a route, begin at the (S)tart point (row & column) provided by the required locations file. From there, find the closest vaccination site to the current location using the distance formula provided in the TSP algorithm (page 3). Once found, move to it – this means that your current coordinates must be updated to those of that closest vaccination site. Every time you visit a new vaccination site, mark that site as Visited, update current location to that site, and use the TSP algorithm to determine the next site that is nearest to your current location and that has not yet been Visited. After each move, you must check all remaining unvisited vaccination sites to determine which is nearest to your current location. Once all vaccination sites have been visited, your driver travels to the (E)nd point. 作为二二疫苗™驱动程序的调度程序,实现最近邻算法。要确定路线,请从要求位置文件提供的(S)倾斜点(行和列)开始。从那里,使用TSP算法中提供的距离公式找到离当前位置最近的疫苗接种地点(第3页)。一旦找到,移到它,这意味着当前坐标必须更新到最近的疫苗接种站点的坐标。每次您访问一个新的疫苗接种站点时,将该站点标记为已访问,更新当前位置到该站点,并使用TSP算法确定最近且尚未访问的下一个站点。每次移动后,您必须检查所有未访问的疫苗接种站点,以确定哪个离您当前位置最近。一旦访问了所有的疫苗接种地点,你的司机将前往(E)点。
To establish the driver’s route, you can make use of two loops:
- The inner loop should iterate through the locations not yet visited to determine which vaccination site is the closest to the current location – solely based on the nearest neighbor algorithm. 内环应该迭代尚未访问过的位置,以确定哪个疫苗接种地点离当前位置最近——仅基于最近邻算法。
Really Important Note
It may be that when you are deciding on the next vaccination site to visit, there is more than one vaccination site at exactly the same distance from your current location. If this case is true, then you must choose the site with the lower ID value. 它可能是,当你决定访问下一个疫苗接种地点时,有不止一个疫苗接种地点与你现在的地点有完全相同的距离。如果此情况为真,则必须选择ID值较低的站点。
- The outer loop iterates while there are sites that have not yet been visited. It should also be the place where you output the necessary text to txtindicating where you are going to next. Refer the description of the route.txt file and the test cases for more details. 当有尚未访问的站点时,外部循环会进行迭代。它也应该是你输出必要文本的地方。txt指示你下一步的位置。有关详细信息,请参考路由。txt文件和测试用例的说明。
Program Description
In general, you need to write a C program that:
- Reads in the locations and vaccination site names
- Processes the locations and vaccination site names
- Determines the route to take
- Outputs a file summarizing the route
- 阅读这些地点和疫苗接种地点的名称
- 处理这些地点和疫苗接种站点的名称
- 确定要采取的路线
- 输出一个总结该路线的文件
Note: The above list can be considered to be the start of a program design for this project. You will be required to submit a full program design as part of this project. Refer to the Program Design Section for more details on what you need to submit. Refer to the Project 4 Overview slides on Moodle for some inspiration on how to structure your program. These will be posted over the weekend.注:上述列表可视为本项目程序设计的开始。您将需要作为本项目的一部分提交一个完整的项目设计。有关您需要提交的内容的更多详细信息,请参阅程序设计部分。有关如何结构您的项目,请参考项目4Moodle概述幻灯片。这些信息将在周末发布。
Terminology
In this program description, the following terms will be used:
- Input Files:Files that will be used by your function, such as a .txt
- Output Files:Files that will be produced by your function, such as a .txt
Required Program Computer Science代写
There is one required program file for this project. The C file for this program must be named DiDiVaccine.c. There are no helper functions required for this project, but I highly recommend you create some to help abstract away some of the complexity of this project (that is, to break up your code, or modularize, in order to directly test small pieces of it). You can copy/paste your helper functions into a separate code file to test them one-by-one (be sure to call them from the main() to test!). 此项目有一个必需的程序文件。此程序的C文件必须命名为DiDiVaccine.c。这个项目不需要辅助函数,但我强烈建议您创建一些来帮助抽象这个项目的一些复杂性(也就是说,分解代码或模块化,以直接测试它的小部分)。您可以将助手函数复制/粘贴到一个单独的代码文件中,以逐个测试它们(一定要从主()调用它们来进行测试!)。
Input Files
There are two input data files that your code must read: 1) a file containing the locations your DiDiVaccine™ driver is required to visit, and 2) a file containing the names of the vaccination sites with their ID numbers. These two files are defined in the next sections. 您的代码必须读取两个输入数据文件:1)一个包含DiDiViccine™驱动程序需要访问的位置的文件,以及2)一个包含疫苗接种站点名称及其ID号码的文件。这两个文件将在下一个部分中进行定义。
Snapshots of each file can be seen in the Appendix; these input files are also located in the Project 4 Moodle folder.每个文件的快照可在附录中看到;这些输入文件也位于项目4Moodle文件夹中。
Recall that the names of the input files will be entered by the user via the terminal. Refer back to Get Filenames for details of how to handle this. 记住,用户将通过终端输入输入文件的名称。有关如何处理此问题的详细信息,请参阅返回以获取文件名。
Required Locations File
This file contains information about the locations your DiDiVaccine™ driver must visit. The first line in the file gives the number of rows and the number of columns, respectively, defining the limits of the grid in which you will dispatch. Lines two and three contain the coordinates of your starting point and the ending point, in that order. Next is a list of vaccination site locations to which the vaccines must be delivered. 此文件包含有关DiDi疫苗™驱动程序必须访问的位置的信息。文件中的第一行分别给出行数和列数,以定义了要发送的网格的限制。第2行和第三行按该顺序包含起点和终点的坐标。接下来是必须交付疫苗的疫苗接种地点的列表。
The data about each vaccination site location include row and column coordinates, a symbol representing the location, and a location ID number. Some vaccination sites may have coordinates that lie outside the grid dimensions – refer back to Create Location Grid for how you should handle this. 关于每个疫苗接种地点位置的数据包括行和列坐标、表示位置的符号和位置ID号。一些疫苗接种站点可能的坐标位于网格维度之外-请参阅“创建位置网格”以了解如何处理此问题。
You are guaranteed that the input will be structured correctly and all IDs given will be unique integer numbers. You are also guaranteed that the start point and the end point shall be within range and that the vaccination sites to be visited are not at the same location as either the start point or the end point. 您保证输入的结构将正确,并且给出的所有ID都将是唯一的整数。您还保证起点和终点应在此范围内,并且待访问的疫苗接种地点与起点或终点的位置不相同。
INPUT: <location_file>.txt Computer Science代写
<Number of Grid Rows> <Number of Grid Columns>
<Starting Row> <Starting Column>
<Ending Row> <Ending Column>
<Vac Site 1 Row> <Vac Site 1 Column> <Vac Site 1 Symbol> <Vac Site 1 ID>
<Vac Site 2 Row> <Vac Site 2 Column> <Vac Site 2 Symbol> <Vac Site 2 ID>
…
The values in this file are explained below:
- <Number of Grid Rows>– the number of rows there are in your grid
- <Number of Grid Columns>– the number of columns there are in your grid
- <Starting Row>– the starting point’s row number (not index number)
- <Starting Column>– the starting point’s column number (not index number)
- <Ending Row>– the ending point’s row number
- <Ending Column>– the ending point’s column number
- <Vac Site Row>– the vaccination site’s row number
- <Vac Site Column>– the vaccination site’s column number
- <Vac Site Symbol>– the symbol associated with the vaccination site’s location (this will always be one character in length)
- <Vac Site ID>– a unique integer ID that is associated with the vaccination site
Vaccination Site Names File Computer Science代写
This file contains a list of vaccination site IDs and names. The file will contain the same location IDs given in <location_file>.txt, not necessarily in the same order and not only these location IDs, followed by the name of each location. During transmission, this file has been corrupted. It contains some meaningless pairs of Y’s which require removal. You must also replace underscores (“_”) in the names with spaces in your output file. You are guaranteed that you will have the correct number of ID and name pairs in this file that correspond to valid vaccination sites in the location file. 此文件包含疫苗接种站点ID和名称的列表。该文件将包含在<location_file>。txt中给出的相同的位置ID,但不一定是按照相同的顺序,而且不仅是这些位置ID,后面跟着每个位置的名称。在传输期间,此文件已损坏。它包含了一些需要删除的毫无意义的Y对。您还必须用输出文件中的空格替换名称中的下划线(“_”)。您保证此文件中与位置文件中有效疫苗接种站点对应的ID和名称对。
INPUT: <name_file>.txt
<Vac Site 1 ID> <Vac Site 1 Name>
<Vac Site 2 ID> <Vac Site 2 Name>
…
The values in this file are explained below:
- <Vac Site ID>– a unique ID that is associated with the vaccination site
- <Vac Site Name>– a name that is a contiguous set of characters (i.e. no spaces)
Output File Computer Science代写
There is one output file for this program: route.txt. This file contains a grid map of the route and a list of all the vaccination sites that have been visited in the correct order based on the rules below. Below is the structure for the file: 此程序有一个输出文件:route.txt。此文件包含路线的网格地图,以及根据以下规则按正确顺序访问过的所有疫苗接种站点的列表。以下是该文件的结构:
OUTPUT: route.txt
<Map>
Start at <Starting Row> <Starting Column>
Go to <Vac Site Name> at <Vac Site Row> , <Vac Site Column>
…
End at <Ending Row> <Ending Column>
The values in this file are explained below (see the test cases in the Appendix for an example of route.txt) : 此文件中的值说明如下(有关route.txt的示例,请参见附录中的测试示例):
- <Map>– the grid with all the valid vaccination sites’ symbols on it
- <Starting Row>– the starting point’s row number
- <Starting Column>– the starting point’s column number
- <Vac Site Name>– a name that is a contiguous set of characters
- <Vac Site Row>– the vaccination site’s row number
- <Vac Site Column>– the vaccination site’s column number
- <Ending Row>– the ending point’s row number
- <Ending Column>– the ending point’s column number
Hints for a Successful Program Computer Science代写
We highly recommend using structs to store the vaccination site data. Structs are a good choice because each vaccination site has the same set of data (name, ID, location, etc.) and because one naturally thinks about the vaccination site first and then all the data associated with that vaccination site (as opposed to thinking of a bunch of names, then a bunch of IDs, then a bunch of row numbers, etc.). An array of structs will conveniently store your vaccination site data for you to use later in your program. 我们强烈建议使用结构来存储疫苗接种站点数据。结构是一个很好的选择,因为每个疫苗接种站点都有相同的数据集(名称、ID、位置等)。因为人们自然会首先考虑疫苗接种站点,然后是考虑与疫苗接种站点相关的所有数据(而不是考虑一堆名字,然后是一堆ID,然后是一堆行号,等等)。一组结构将方便地存储您的疫苗接种站点数据,以便您稍后在您的程序中使用。
Recall that when you write functions that pass in large data structures, like arrays and structs, you should use pass-by-address. Refer back to the lecture slides on when to use pass-by-value, pass-by-address, and const pass-by-address. 请记住,在写入传递大型数据结构的函数,如数组和结构时,应该使用传递地址。请参考何时使用传递值、传递地址和常传递地址的讲座幻灯片。
There are many, many different ways to successfully approach this project. We encourage you to develop a good program design before coding anything. This can be pseudocode, a flowchart, or a combination of the two. If you are stuck trying to get started, refer back to what we’ve covered in lectures and labs: char array manipulation, arrays, structs, arrays of structs, functions, etc. 成功完成这个项目的方法有很多很多不同。我们鼓励您在编写任何东西之前,先开发一个好的程序设计。这可以是伪代码、流程图或两者的组合。如果您一直试图开始,请参考我们在讲座和实验室中介绍的内容:字符数组操作、数组、结构、结构数组、函数等。 Computer Science代写
Submission and Grading
Project 4 has two deliverables: the program design and the DiDiVaccine.c file. Grades are broken down into 3 categories: the program design, functionality, and the style and comments. 项目4有两个可交付成果:程序设计和DiDiVaccine.c文件。等级分为三个类:程序设计、功能、风格和评论。
Project 4 will be graded in three parts. First, we will evaluate the general completeness of your program design that is submitted to Moodle and will provide a maximum score of 10 points. Second, the functionality of your program will be evaluated for your submission. Third, we will evaluate your submission for style and commenting and will provide a maximum score of 10 points. Thus, the maximum total number of points on this project is 120 points. Each test case is worth the same amount. Some test cases are public (meaning you can see your output compared to the solution as well as the inputs used to test your submission) while many are private (you are simply are told pass/fail and the reason). The breakdown of points for each category is described in the next sections. 项目4将分为三部分进行分级。首先,我们将评估提交给Moodle的项目设计的总体完整性,并提供最高得10分。其次,将为您的提交内容评估程序的功能。第三,我们将评估您提交的风格和评论,并提供最高10分。因此,本项目的最大积分总数为120分。每个测试用例的价值都是相同的。一些测试用例是公开的(这意味着您可以看到您的输出与解决方案的比较,以及用于测试提交的输入),而许多测试用例是私有的(您只是被告知通过/失败和原因)。在下一节中描述了每个类别的点的细分。
Program Design Computer Science代写
Maximum Score: 10 points
This project requires a complex program that performs two major tasks that need to be broken down into several smaller sub-tasks. Therefore, a program design document is a required deliverable. A good program design helps to find logic errors (those are the hard ones to debug, remember!) and helps immensely when describing your program to someone. Dr. E will ask to see this program design document if you ask for help debugging your program.这个项目需要一个复杂的程序,它执行两个主要任务,需要分解为几个较小的子任务。因此,程序设计文件是必需的可交付文件。一个好的程序设计有助于找到逻辑错误(记住,这些是很难调试的!)并且在向某人描述你的程序时会有极大的帮助。如果您要求帮助调试程序,E博士会要求查看这个程序设计文档。
Project 3 used a flowchart. You may also use pseudocode for program designs. You may use either of these approaches, both of these approaches, a mixture of these approaches, or something else entirely. The choice is yours. If you choose to use a flowchart at any point, you can make a flowchart using pen and paper, PowerPoint or any other programs that have the flowchart shapes built-in. If you choose to use pseudocode at any point, make sure that it is a detailed list of steps/algorithms/abstractions (helper functions). 项目3使用了一个流程图。您也可以使用伪代码进行程序设计。您可以使用这两种方法,这两种方法,这些方法的混合物,或者完全使用其他方法。您可以自行选择。如果选择在任何点使用流程图,则可以使用笔和纸、PowerPoint或任何具有内置流程图形状的其他程序来创建流程图。如果您选择在任何时候使用伪代码,请确保它是步骤/算法/抽象(辅助函数)的详细列表。
Your program design should show how you are breaking down the major tasks into smaller subtasks (consider how the Program Tasks section is broken into subsections for inspiration). There must be a “Start” and an “End” to the program design, with a clear control flow from start to end, but there should not be actual code in the program design (pseudocode is okay). For example, if you are removing an element from a vector within a loop, you have to show this in the program design but not write the actual code you would use. If you have questions, send your program design to Dr. E and get feedback! 您的程序设计应该展示如何将主要任务分解为较小的子任务(考虑如何将程序任务部分分解为子部分以获得灵感)。程序设计必须有“开始”和“结束”,从头到尾有清晰的控制流,但程序设计中不应应有实际代码(假代码可以)。例如,如果要从循环中的向量中删除元素,则必须在程序设计中显示它,但不编写要使用的实际代码。如果你有问题,把你的项目设计发送给E博士并得到反馈!
Submitting Your Program Design Computer Science代写
Your program design will be submitted via Moodle. It will be graded for general completeness and quality, i.e. that the major steps required are documented and described and that the design is clear and easy to understand and not scribbled on the back of a napkin with a sharpie. It will not be graded on style, graphic design, or “prettiness,” so don’t spend a lot of time worrying about your color scheme! 您的程序设计将通过Moodle提交。它将根据一般的完整性和质量进行分级。所需的主要步骤被记录和描述,设计清晰和容易理解,不在餐巾纸背面乱写。它不会根据风格、平面设计或“漂亮”进行分级,所以不要花很多时间担心你的配色方案!
Save your program design as a .pdf or .ppt file with Name, Partner Name (or “none”), and Date Submitted at the top. Go to Moodle and upload your file. Unlike Project 3, if you are working with a partner, you only need to submit one program design file for you both! If you are working with a partner, be sure you both contribute to this program design file!将程序设计保存为。pdf或。ppt文件,顶部包含“名称”、“合作伙伴名称”(或“无”)和“提交日期”。去Moodle并上传你的文件。与项目3不同,如果你正在与合作伙伴合作,你只需要为你双方提交一个程序设计文件!如果您正在与合作伙伴合作,请确保您俩都贡献了这个程序设计文件!
Note on Quality of Submission Computer Science代写
Your program design must be a document that is of professional quality. This means text is typed or clearly written and flowcharts are done using some kind of software program (PowerPoint, Microsoft Word, etc.). Handwritten submissions must be CLEARLY written and scanned into an electronic document (.pdf).您的程序设计必须是具有专业质量的文件。这意味着文本被输入或清晰地编写,流程图是使用某种软件程序(PowerPoint、微软Word等)完成。手写文件必须清楚书写并扫描成电子文件(。pdf)。
Functionality
Maximum Score: 100 points
Submit your DiDiVaccine.c file to Moodle for final grading. As with previous projects, you may submit your code to Dr. E for feedback. You will be provided with a final score (out of 100 points) as well as information on a subset of its test cases. 提交你的DiDiVaccine.c文件给Moodle进行最终分级。与以前的项目一样,您可以将您的代码提交给E博士以获得反馈。您将获得最终分数(100分中)以及有关其测试用例子集的信息。
You are limited to a total of 10 submissions on this project. I highly recommend that you develop tests to find scenarios where your code might not produce the correct results prior to sending it to me. 此项目只能提交10份文件。我强烈建议您开发测试,以找到在发送代码之前可能不会产生正确结果的场景。
You will receive a score for the functionality portion equal to the score of your best submission. Your Moodle submission will be the code that is style graded.
Please refer to the syllabus for more information regarding partner groups.
Style and Commenting Computer Science代写
Maximum Score: 10 points
2pts – Each submitted file has English Name, Partner English Name (or “none”), Project Name, Due Date included in a comment at the top
2pts – Comments are used appropriately to describe your code (e.g. major steps are explained)
2pts – Indenting and whitespace are appropriate (including functions are properly formatted)
2pts – Variables are named descriptively
2pts – Other factors (variable names aren’t all caps, etc…)
Appendix: Test Cases
Here are two test cases for you to use in designing, writing, and testing your code.
Test Case 1 – Shantou Computer Science代写
Command Prompt:
C:\Users\Eric\Project4>gcc DiDiVaccine.c -o DiDiVaccine
C:\Users\Eric\Project4>DiDiVaccine
Enter Locations Filename: location_1_test.txt
Enter Names Filename: name_1_test.txt
6341 out of range – ignoring
7632 out of range – ignoring
8751 out of range – ignoring
C:\Users\Eric\Project4>
INPUT: location_1_test.txt
5 14
1 2
2 1
1 4 T 7925
3 12 P 2523
4 16 F 6341
4 8 C 2340
-2 2 L 7632
5 17 D 8751
5 1 V 5555
3 6 R 9349
2 6 W 5125
4 6 Z 1112
5 4 2 4318
INPUT: name_1_test.txt Computer Science代写
2340 SUMC_Campus
4318 SUMC_No.2_Affiliated_Hospital
8751 Shan’an_Driving_School
5555 Tiangang_Village_Center
9349 Shantou_Ren’ai_Hospital
2523 Longhu_People’s_Hospital
7632 Longjin_Community_Clinic
7925 Tuopu_Community_Clinic
5125 Wanda_Plaza
6341 F16
1112 Zhongshan_Park
OUTPUT: route.txt
.S.T……….
E….W……..
…..R…..P..
…..Z.C……
V..2……….
Start at 1 2
Go to Tuopu Community Clinic at 1 4
Go to Wanda Plaza at 2 6
Go to Shantou Ren’ai Hospital at 3 6
Go to Zhongshan Park at 4 6
Go to SUMC Campus at 4 8
Go to SUMC No.2 Affiliated Hospital at 5 4
Go to Tiangang Village Center at 5 1
Go to Longhu People’s Hospital at 3 12
End at 2 1
Test Case 2 – Mysteria Computer Science代写
TERMINAL:
C:\Users\Eric\Project4>gcc DiDiVaccine.c -o DiDiVaccine
C:\Users\Eric\Project4>DiDiVaccine
Enter Locations Filename: location_2_test.txt
Enter Names Filename: name_2_test.txt
7179 out of range – ignoring
C:\Users\Eric\Project4>
INPUT: location_2_test.txt
14 12
13 10
1 3
4 12 T 4497
13 3 P 7932
2 8 Q 7999
-1 -1 X 4301
12 10 L 6473
5 4 A 7543
14 10 T 1543
INPUT: name_2_test.txt Computer Science代写
2573 ZevYYon
5821 YYYougo
8134 Hello_Goodbye_YMCYYA
0981 Bad_NeYYws_Bears_Community_CentYYer
9812 GoodYYness
7179 Homeward_Bound_YY
7831 PheboYYs
OUTPUT: route.txt
……………
…E………T.
……………
………..T…
……………
…….A…….
……………
.Q……….L..
……………
…………S..
……………
…………P..
……………
……………
……………
Start at 10 13
Go to Phebos at 12 13
Go to Hello Goodbye YMCA at 8 13
Go to Yougo at 6 8
Go to Bad News Bears Community Center at 4 12
Go to Zevon at 2 14
Go to Goodness at 8 2
End at 2 4